JavaScript code snippets
Set focus in forms so the
cursor automatically appears in the first field
- Know the names of the form and the field you want the cursor to appear in.
- Add the following code just after the </form> tag at the end of the
form, replacing "formname" with the name of your form and "fieldname" with
the ID of your field.
<script>
<!--
document.formname.fieldID.focus();
</script>
Select field contents
when focus moves to field
Add the following code to the tag that defines the field in question.
onFocus="this.select();"
The complete tag will look something like this:
<input onFocus="this.select();" type="text" name="MyInputField"
size="20" value="Enter name here">
Displaying and hiding instruction text in a text field
You may want to include instruction text within a text field as shown below.
When
you do this, the field must be within a table of the class "Form" and
the text box must include the class "InstructionText" to
make the instruction text italic and gray.
<input class="MarginLeft2px Width200px InstructionText" name="EntityName" id="EntityName" type="text" value="Type all or part
of name" onFocus="this.select();" onKeyUp="checkClass('EntityName','InstructionText');
return false;" onBlur="checkBlank('EntityName','InstructionText');
return false;">
Three events are used:
- onFocus="this.select();", which selects all the field contents when the
field is selected
- onKeyUp calls the function checkClass when the user types within the field.
checkClass looks to see if the "InstructionText" class is in use
and, if so, removes it so that user-entered text is black and not italic.
onKeyUp passes the id of the text field and the InstructionText class.
- onBlur calls the function checkBlank when the user moves elsewhere. If
the user has left the field blank, the instruction text reappears and the
InstructionText class is reapplied. onBlur also passes the id of the text
field and the InstructionText class.
The script, which is placed in the <head> section, is shown below.
<script>
function checkClass(myID,cls) {
var ele = document.getElementById(myID);
if (hasClass(ele,cls)) {
var reg = new RegExp('(\\s|^)'+cls+'(\\s|$)');
ele.className=ele.className.replace(reg,' ');
}
else {}
return false;
}
function hasClass(ele,cls) {
return ele.className.match(new RegExp('(\\s|^)'+cls+'(\\s|$)'));
}
function checkBlank(myIDagain,cls) {
var ele = document.getElementById(myIDagain);
if (ele.value == "") {
ele.value = "Type all or part of name";
ele.className += " "+cls;
}
else {}
return false;
}
</script>
Create a browse file field
Add the following code:
<input type="file" name="datafile" size="40"/>
Create a link that goes nowhere (for
prototypes only)
Use the following code:
<a href="javascript:void(0)" onClick="return
false;">link text</a>
Go to another URL on click
Add the following code to the tag containing the object (e.g., a button) or
to the Javascript.
In-page anchor: <input
type="button" name="CheckWork" value="Check Work" onclick=window.location="#SectionB";>
Another page: <input
type="button" name="Next" value="Next Page" onclick="document.location.href='Assign
Delegates after Delegate Added.htm'">
Note: This does not work when the input type="submit"
Call a JavaScript function: <input
type="radio" name="Q2" value="No"
onclick="question2()">
Here's one JavaScript function:
<script>
function question2() {
window.location="#SectionB";
}
</script>
Here's another:
<script>
function GotoSummaryPage() {
location.href = "Summary.htm";
}
</script>
Open a popup window or dialog
on click
Here's an example of the code for a link that calls a popup window.
<a href="_Link%20Example%20Results%20Page.htm" target="_LinkExampleResults"
onclick="window.open(this.href,
this.target, 'width=700,height=650,scrollbars=yes,resizable=yes'); return
false;">Link Example Results</a>
Here's an example of the code for a link that calls a modal popup dialog.
Modal popup dialogs do not allow users to return to the original window without
first closing the dialog. Be sure to include the "return false;" it prevents
the link included in the href parameter from actually being called.
onclick="window.showModalDialog('Regional Fraud Audit Assistance Leaders.htm',window,'dialogWidth:700px;
dialogHeight:500px; status:no'); return false"
Add the following code to the tag containing a button calling a modal
dialog.
onclick="window.showModalDialog('Successful%20Save.htm',window,'dialogWidth:600px;
dialogHeight:250px; status:no');"
If the onclick is part of a button that already has an onclick behavior, add
it to the behavior as shown below.
onclick="window.showModalDialog('Successful%20Save.htm',window,'dialogWidth:600px;
dialogHeight:250px; status:no');
"
It's a good practice to give users a way to close and print the new window.
Provide them with a link with these onclick parameters for closing and printing,
respectively:
onclick="window.close(); return false"
onclick="window.print(); return false"
Expand
and collapse rows in a table
This script will let you use expand/collapse or a link to display and hide
rows within a table.
The JavaScript below goes in the page's <head> tag. This example is
to show and hide submenu items in the left navigation. The parameters being
passed to the script (image and row) are the id for the plus or minus image
and the id for the row that will be displayed or hidden.
<script>
function viewMenu(rowSubmenu,rowImage) {
var row = document.getElementById(rowSubmenu);
var image = document.getElementById(rowImage);
if (row.style.display == 'none') {
row.style.display = '';
image.src = "images/Tree/LeftNavCollapse.gif";
}
else {
row.style.display = 'none';
image.src = "images/Tree/LeftNavExpand.gif";
}
return false;
}
</script>
Here is the tag for the link that enclosesthe plus/minus image and the image
itself. Note the unique id, the title text, and the return false in the onclick
event. The onclick passes the image and row parameters (their unique ids)
to the JavaScript function, viewMenu.
<a id="rowThreeLink" href="bogus26.htm" onClick="viewMenu('rowThreeSubmenu','rowThreeImage');
return false;" title="Click to view or hide items one level down"><img
id="rowThreeImage" src="images/Tree/LeftNavExpand.gif" border="0"></a>
Here is the tag for the row. Note the row style is set to display:none and
the row has a unique id.
<tr id="rowThreeSubmenu" style="display:
none;">
Here's a different example where both an image and a link displays
or hides the row and the link text changes from "show" to "hide." Note that "return
false" must
be part of the onclick and the link should have an ID, in this case, "changeText." The
Javascript that changes the text from show to hide is this: document.getElementById("changeText").innerHTML="Hide
Guidance for Engagement and Control Environment Risk Factors";
<a href="Bogus.htm" onclick="viewGuidance('Image_EngagementAndControlEnvironmentRiskFactors','Row_EngagementAndControlEnvironmentRiskFactors');
return false;" title="Click to show or hide guidance" id="changeText">View
Guidance for Engagement and Control Environment Risk Factors</a>
Display and hide
an image
You may have an image in an HTML document that you only want displayed some
of the time. Put the image in the document, give it a name, and set the style
for display = none (e.g., <img border="0" src="images/YouAreHere.gif" alt="Current
Question"
style="display: none; padding-top: 3px;" name="Question5Arrow" width="24" height="14">
).
The script that will make this arrow appear is:
window.document.Question5Arrow.style.display = "";
It might be in a function, as shown above, or an onclick, e.g., <input
type="button" name="ShowArrow" value="Show Arrow"
onclick=window.document.Question5Arrow.style.display = "";>
.
Caution: Once the arrow appears, you might want it to disappear
whenever you click something else. So you'll probably write a hideArrow
function and call it whenever anything else on the page gets clicked. See Call
a Function from Another Function on this page for instructions on how to
do this.
<script>
function hideArrows() {
window.document.Question5Arrow.style.display = "none";
return
}
</script>
Make the current date appear
Paste the following code where you want the current date to appear, e.g.,
August 25, 2004.
<script>
var d=new Date()
var monthname=new Array("January","February","March","April",
"May","June","July","August",
"September","October","November","December")
document.write(monthname[d.getMonth()] + " ")
document.write(d.getDate() + ", ")
document.write(d.getFullYear())
</script>
Make a radio button display a row
and move the cursor to a field in the row
Call the function with an event handler like this, where the parameters being
passed is a radio button group name, the table row id (<tr id="Q4aMitigationRow"
style="display: none;">), and the name of field that the cursor
will appear in (<textarea rows="5" id="Q4aMitigationComments" cols="65" class="ContentFont" name="Q4aMitigationComments"
onfocus="this.select();">You selected GTNR or MGTNR. Enter your
mitigation strategy here.</textarea>). Note that when the cursor arrives
in the field the prompt text will be selected so the user can beginning typing
without erasing it (the onfocus="this.select();"
in the textarea field).
<input type="radio" name="Q4a" value="NR"
onclick="mitigationRow(Q4a,Q4aMitigationRow,Q4aMitigationComments)">
The function will look like this:
<script>
function mitigationRow(question,row,commentsField) {
if ((question[1].checked == true) || (question[2].checked == true)) {
row.style.display = "";
commentsField.focus();
}
else {
row.style.display = "none";
}
}
</script>
This script receives the parameters. Because we need to find which of three
radio buttons are checked (the possibilities are [0],[1], and [2]), the if statement
asks if the second one is checked (question[1].checked == true) or (||) the
third one is checked (question[2].checked == true). If either the second or
third button is checked, the row with the field should be displayed and the
cursor should move to that field. If the first button is selected, the row should
not display. The function is called by the same onclick for all three radio
buttons.
Call a function from another function
Rather than putting one bit of code in a whole bunch of scripts, write it
once and call it from the other scripts.
Here's the calling function which, depends on what radio buttons are selected,
enables or disables other questions. The calling function is simply the name
of the function being called, e.g., hideArrows(); or question3Disabled();.
function question1(ErrorRow) {
hideArrows();
if (Form1.Q1[0].checked == true) {
ErrorRow.style.display = "";
hideArrows();
question2Disabled();
question3Disabled();
question4Disabled();
question5Disabled();
}
else {
ErrorRow.style.display = "none";
question2Enabled();
question5Enabled();
if (Form1.Q2[0].checked == true) {
question3Disabled();
question4Disabled();
}
else if (Form1.Q3[0].checked == true) {
question3Enabled();
question4Disabled();
}
else {
question3Enabled();
question4Enabled();
}
}
}
Here's one of the functions being called. The return sends the script
back to the function that called it.
function question3Disabled() {
Q3AnswerText.className = "NotAvailable";
Form1.Q3[0].disabled = true;
Form1.Q3[1].disabled = true;
Q3Text.className = "Question NotAvailable";
return
}
The function is actually disabling the two radio buttons in question 3 (Form1.Q3[0]
and Form1.Q3[1] where Form1 is the name of the form, Q3 is the name of the radio
button group (all radio buttons in a group have the same name) and [0] is the
first radio button in the group and [1] is the second radio button in the group.
It's also changing the class of the text for several table cells (e.g., <td
valign="top"
nowrap class="Available" id="Q3AnswerText">) from Available
(defined as black in the cascading style sheet) to NotAvailable (defined as
gray).
Single click for checking/unchecking
multiple checkboxes
This is useful when you want the user to carry out some task on either all
or none of the items that you present.
<script>
function modify_boxes (total_boxes){
for ( i=0; i < total_boxes; i++ ){
if (document.Application.CheckAll.checked == true ) {
document.Application.chkboxarray[i].checked=true;
}
else {
document.Application.chkboxarray[i].checked=false;
}
}
}
</script>
<body>
<form name="Application" id="Application">
<input type="checkbox" name="CheckAll" value="SelectAll" class="DropShadow" onclick="modify_boxes
(3)">
<input type="checkbox" name="chkboxarray" value="AERS"><br>
<input type="checkbox" name="chkboxarray" value="Consulting"><br>
<input type="checkbox" name="chkboxarray" value="Tax"><br>
</form>
</body>
Note: The VALUE tags for the checkboxes seem to have no use. But it
is required to differentiate between the checkboxes when you submit the form
to a server-side program. You can differentiate between the checked boxes by
giving different values to each checkbox.
Another script for this, from ASP
Warrior, follows. This one does not require you to know how many checkboxes
there are:
<SCRIPT LANGUAGE="JavaScript">
function checkAll(field)
{
for (i = 0; i < field.length; i++)
field[i].checked = true ;
}
function uncheckAll(field)
{
for (i = 0; i < field.length; i++)
field[i].checked = false ;
}
</script>
<form name="myform" action="checkboxes.asp" method="post">
<b>Your Favorite Scripts & Languages</b><br>
<input type="checkbox" name="list" value="1">Java<br>
<input type="checkbox" name="list" value="2">Javascript<br>
<input type="checkbox" name="list" value="3">Active
Server Pages<br>
<input type="checkbox" name="list" value="4">HTML<br>
<input type="checkbox" name="list" value="5">SQL<br>
<input type="button" name="CheckAll" value="Check
All"
onclick="checkAll(document.myform.list)">
<input type="button" name="UnCheckAll" value="Uncheck
All"
onclick="uncheckAll(document.myform.list)">
<br>
</form>
Filling the values of a dropdown
menu depending on the selection in another menu
When the user selects an entry in the first dropdown menu, the relevant date
in the second menu appears. The source for the code is http://www.geocities.com/paikiran/articles/9tips.html.
var tennisplayers= new Array("Safin", "Andre Agassi", "Pete
Sampras", "Anna Kournikova", "Martina Hingis");
var cricketplayers = new Array("Sachin Tendulkar", "Steve Waugh", "Brian Lara", "Sir
Don Bradman");
function set_player() {
var select_sport = document.myform.sport;
var select_player = document.myform.player;
var selected_sport = select_sport.options[select_sport.selectedIndex].value;
select_player.options.length=0;
if (selected_sport == "tennis"){
for(var i=0; i<tennisplayers.length; i++)
select_player.options[select_player.options.length]
= new Option(tennisplayers[i]);
}
if (selected_sport == "cricket") {
for(var i=0; i<cricketplayers.length; i++)
select_player.options[select_player.options.length]
= new Option(cricketplayers[i]);
}
}
<BODY>
<FORM NAME="myform" METHOD="POST">
Sport
<SELECT NAME="sport" onChange="set_player()">
<OPTION VALUE="tennis">-----
<OPTION VALUE="tennis">Tennis
<OPTION VALUE="cricket">Cricket
</SELECT>
</FORM>
</BODY>
Go to another URL based on the value
of a dropdown options
When the user selects an entry in a dropdown menu, another page appears in
the browser window, depending upon which option is selected.
In the example below, the dropdown is within a form with id="Application" and
the dropdown has id="statementDates".
<script>
function changeYear() {
if (Application.statementDates.options[Application.statementDates.selectedIndex].value
== "FY06") {
document.location.href="Financial Statements for Current
FY.htm";
}
else if (Application.statementDates.options[Application.statementDates.selectedIndex].value
== "FY05") {
document.location.href="Financial Statements for Past
FY.htm";
}
else {
document.location.href="Financial Statements for Two
FYs Ago.htm";
}
}
</script>
The dropdown tag looks like this: <select size="1" id="statementDates" class="EditItem" onChange="changeYear();">
.
A typical option within the select tag looks like this: <option
value="FY05">FY05</option>
.
Populating a text field and
table cell contents based on entries in multiple dropdowns (includes
JavaScript equals, does not equal, and, or, if, else if; tests whether multiple
conditions are met; and builds a text string)
This code:
- Provides a statement name (that the user can then edit) based on the user's
selections for Report ID, Fiscal Year, and text entry for Statement Date. For
example, if the user selects 330_A: Return on Investment and FY07 and types
7/1/2006, the statement name will be Return on Investment FY07 (7/1/2006).
If the user makes no entry for the statement date, the statement name will
be Return on Investment FY07.
- Populates the contents of a table cell with a category based on the report
ID the user selects. The user cannot edit the category.
Names and ids used in the script and HTML:
- Form: Application
<form name="Application" id="Application">
- Function: nameStatement()—called onChange for each of two dropdowns
and onKeyUp for the text field
- Dropdown 1: idDropdown—dropdown with possible statement names
<select size="1" name="idDropdown" id="idDropdown" class="EditItem" onChange="nameStatement();">
- Dropdown 2: FiscalYear—dropdown with possible fiscal years
<select size="1" class="EditItem" name="FiscalYear" id="FiscalYear" onChange="nameStatement();">
- Text Cell for Date : StatementDate—text field for entry of statement
dates—note that the function is executed onKeyUp so that as the user
types, the statement name is updated
<input class="EditItem" style="WIDTH:
90px;" name="StatementDate" id="StatementDate" value="mm/dd/yyyy" onFocus="this.select();" onKeyUp="nameStatement();">
- Text field: statementName
<td colspan="3" class="TextDisplayCell"><input
type="text" name="statementName" id="statementName" class="EditItem" style="width:
450px;"></td>
- Table cell: categoryName
<td class="TextDisplayCell" id="categoryName"></td>
The form is shown below:
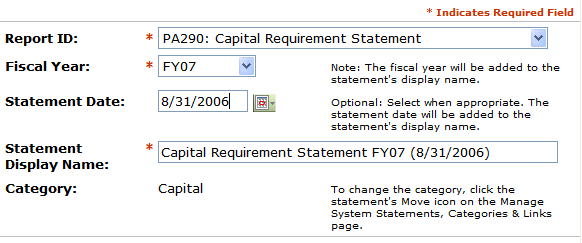
The script is shown below. Each if statement determines whether the selected
report id matches the value within the if and tests for whether or not the period
end date has been entered (tests for the original value of mm/dd/yyyy or a blank
value). When an if statement is executed, it provides a statement display name
and populates the category cell.
<script>
function nameStatement() {
if (Application.idDropdown.options[Application.idDropdown.selectedIndex].value
== "330_A" && (Application.StatementDate.value == "" ||
Application.StatementDate.value == "mm/dd/yyyy")) {
document.getElementById("statementName").value=("Return
on Investment" + " " + Application.FiscalYear.options[Application.FiscalYear.selectedIndex].value);
document.getElementById("categoryName").innerHTML="401(k)";
}
else if (Application.idDropdown.options[Application.idDropdown.selectedIndex].value
== "330_A" && Application.StatementDate.value != "" && Application.StatementDate.value
!= "mm/dd/yyyy") {
document.getElementById("statementName").value=("Return
on Investment" + " " + Application.FiscalYear.options[Application.FiscalYear.selectedIndex].value
+ " (" + Application.StatementDate.value + ")");
document.getElementById("categoryName").innerHTML="401(k)";
}
else if (Application.idDropdown.options[Application.idDropdown.selectedIndex].value
== "330_E" && (Application.StatementDate.value == "" ||
Application.StatementDate.value == "mm/dd/yyyy")) {
document.getElementById("statementName").value=("Estimated
Return on Investment" + " " + Application.FiscalYear.options[Application.FiscalYear.selectedIndex].value);
document.getElementById("categoryName").innerHTML="ROI";
}
else if (Application.idDropdown.options[Application.idDropdown.selectedIndex].value
== "330_E" && Application.StatementDate.value != "" && Application.StatementDate.value
!= "mm/dd/yyyy") {
document.getElementById("statementName").value=("Estimated
Return on Investment" + " " + Application.FiscalYear.options[Application.FiscalYear.selectedIndex].value
+ " (" + Application.StatementDate.value + ")");
document.getElementById("categoryName").innerHTML="ROI";
}
else {
}
}
</script>
The idDropdown is shown below.
<select size="1" name="idDropdown" id="idDropdown" class="EditItem" onChange="nameStatement();">
<option value="None" selected>Select one</option>
<option value="330_A">330_A: Return on Investment</option>
</select>
The FiscalYear dropdown is shown below.
<select size="1" class="EditItem" name="FiscalYear" id="FiscalYear" onChange="nameStatement();">
<option value="" selected>Select one</option>
<option value="FY07">FY07</option>
<option value="FY06">FY06</option>
</select>
The Statement Date text field is shown below.
<input class="EditItem" style="WIDTH:
90px;" name="StatementDate" id="StatementDate" value="mm/dd/yyyy" onFocus="this.select();" onKeyUp="nameStatement();"><img
class="PopcalTrigger" alt="Select from calendar" src="../images/calbtn.gif" border="0" style="vertical-align:
text-bottom; cursor: hand;">
Set a maximum number of characters
in a text area and count them down
This script lets you set a maxlength in a text area field and then use a counter
to let the user see how many characters they have left.
The script is shown below. It also appears in the Simple Form for Entering
Information.htm, located in the _HostedApp directory.
<script>
function ismaxlength(obj){ var mlength=obj.getAttribute? parseInt(obj.getAttribute("maxlength"))
: "";
if (obj.getAttribute && obj.value.length>mlength) {
obj.value=obj.value.substring(0,mlength);
}
CharactersLeft.value=mlength - obj.value.length;
CharactersLeft.innerHTML=CharactersLeft.value;
}
</script>
The text area is shown below.
<tr>
<th>Textarea With <br> Limited Number of<br> Characters:<br></th>
<td><textarea name="textarea" cols="35" rows="5" style="WIDTH:
275px" maxlength="255" onkeyup="return ismaxlength(this)"></textarea><br>
<span class="SmallText" id="CharactersLeft">255</span><span
class="SmallText"> characters left.</span>
</td>
<td class="Example"> </td>
</tr>
Determine the browser width (not including chrome)
Note: This script should immediately follow the <body> tag.
(It has to wait until the body is loaded before it can run.) Therefore, it
should not be called as a body tag onload. The screen width will display in
the browser's status bar (lower left corner).
<body>
<script>
var winwidth = window.innerWidth || document.body.clientWidth;
if (winwidth<=980) {
window.status='The display width is ' + winwidth + ', which is less than or equal
to 980.';
}
else if (winwidth>980 && winwidth<=1030) {
window.status='The display width is ' + winwidth + ', which is greater than 980
and less than or equal to 1030.';
}
else {
window.status='The display width is ' + winwidth + ', which is greater than 1030.';
}
</script> -->